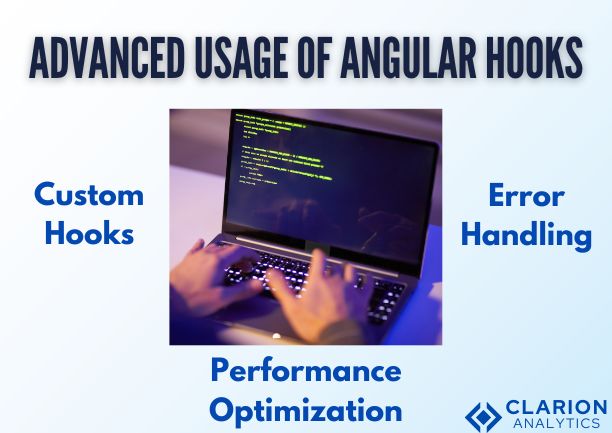
1. Introduction
- 1.1 Overview of Angular Hooks
Among the powerful JavaScript frameworks which have altered the face of modern web application development is Angular hooks. Its architecture is also based on components, scalable, efficient, and maintainable applications. Mainly in this regard, lifecycle hooks play a core role. A lifecycle hook provides developers with the opportunity to run custom code at appropriate points of a component’s lifecycle. This ability gives developers greater control over what is going on in the components and how they interact with other parts of the application.
In Angular hooks , somehow, an element of the application does not appear on your screen just like magic. It goes through several phases: initialization, followed by rendering, then updating, and finally, destruction. Each such stage offers the developer a point to intervene in and custom logic to be run. In Angular, there are a number of hooks which allow developers to “hook” onto those moments and control the application flow.
For example, you may need to initialize data when a component is first created or to do some cleanup when the component is removed. Angular lifecycle hooks make these types of jobs very straightforward and ensure the application runs very efficiently.
- 1.2 Importance of Angular Hooks in Modern Web Development
The high performance and user experiences are needed in applications the larger they become and more complex they get. Angular Hooks serve to let developers reach these goals with detailed control over the lifecycle of a component.
Some of the main reasons as to why Angular Hooks are so important in modern web development are:
- State Management: In lifecycle hooks, this makes the scope to manage the state of components in a predictable manner. The following is an example use of hooks on initializing state at the time of creation of the component or cleaning up the resource used when the component is destroyed.
- Performance Optimization: Hooks allow a developer to precisely control when any logic should be executed. It can thus be used for the improvement of performance within an application, where an example could be delaying heavy computations until the component is fully initialized and ready to go.
- Modularity and maintainability: the code may be organized according to the lifecycle of the components. This brings about better organization of the code, leading to better maintainable applications. It clearly separates concerns and is easier to reason about when a certain logic should be executed.
- Seamless Integration with Services: Dependency injection is achievable in Angular hooks since components can interact with services. Lifecycle hooks make it easy for instance to make service calls at the appropriate time, such as fetching data from an API when a component initializes.
2. What Are Angular Hooks?
- 2.1 Definition and Purpose
Hooks are special callback methods invoked by Angular hooks at specific stages of a component’s lifecycle. Hooks allow developers to tap into key moments in a component’s existence-from creation to property changes, destruction, and so forth. In other words, the lifecycle of an Angular component can be imagined as a series of events. As a component initializes, angular initializes that component and injects all its dependencies. At this stage, a developer may perform initialization of component state, data fetching, or setting up subscriptions. On the other hand, when a component is about to be destroyed, some developers need to clean their resources, for example, event listeners or subscriptions.
Lifecycle hooks by Angular provide a very elegant way to handle such operations.
- 2.2 History and Evolution
Lifecycle hooks have not been introduced with AngularJS. The lifecycle management of AngularJS was rather ad-hoc and therefore relied on custom directives, services to manage component life cycles, and so on. However, with Angular 2 and subsequent releases, lifecycle hooks were introduced to the rescue in making the component’s lifecycle more manageable. In Angular, hooks would provide a simple and uniform means of governing the components’ lifecycle instead of its somewhat more predictable behavior along with fewer complexities than in AngularJS. Angular Hooks do grow in strength and fullness with time. They now support both template-driven and reactive-driven designs, thus enabling the developer to pick the tools that work best for developing responsive and dynamic applications.
3. Types of Angular Hooks
- 3.1 Core Lifecycle Hooks
Angular provides several core lifecycle hooks, and developers can control its behavior. Here’s an in-depth look at each of the key lifecycle hooks.
3.1.1 ngOnInit
The ngOnInit hook gets called after Angular has initialized all data-bound properties of the component. This is, in fact, one of the most frequently used hooks since it’s a very good place for any initialization logic to be put into the component.
Best Practices:
- Use ngOnInit for initialization tasks that depend on input properties or other injected dependencies.
- Heavy processing should be avoided here. Intensive tasks should be offloaded onto another lifecycle hook or async operation.
3.1.2 ngOnChanges
The ngOnChanges hook is called whenever one or more data-bound input properties change. This hook can be very handy when you need to react to changes in the input data or manage any dependency between properties.
Best Practices:
- Use ngOnChanges when there is logic that needs to update when input properties change.
- Avoid very complex logic within this hook because a very complex logic here may suffer from performance.
3.1.3 ngDoCheck
ngDoCheck allows you to implement custom change detection. Even though Angular comes with a change detection mechanism in place, there are times when you might need to detect and respond to changes manually.
Practices Best:
- Use custom change detection only when absolutely necessary because performance will suffer negatively.
- Use Angular’s built-in change detection whenever possible.
3.1.4 ngAfterContentInit
This hook is used in components that use ng-content to project child components into their template. Angular calls this method once after it has projected the external content into the component.
Best Practice:
- Use this hook for any kind of initialization task that depends upon content projection.
- This hook is not recommended for avoiding DOM manipulation from it, since it can influence performance.
3.1.5 ngAfterContentChecked
The ngAfterContentChecked hook is called each time Angular checks for any changes in the projected content. It is useful in detecting change in the content and further performing logic accordingly.
Best Practices:
- Always ensure that the logic within this hook is efficient because it may run very frequently.
3.1.6 ngAfterViewInit
ngAfterViewInit is called after Angular has initialized the view of a component as well as all of its child views. That makes it a great place for doing any DOM manipulations that depend on the existence of the component’s view.
Best Practices:
- Use this hook to perform DOM operations that need to access the view.
- Avoid deep logic within this hook to avoid performance problems
3.1.7 ngAfterViewChecked
ngAfterViewChecked is called after the component’s view (and child views) has been checked for changes. It is useful for detecting changes in the view and responding accordingly.
Best Practices:
- The logic inside this hook should be kept as lightweight as possible.
3.1.8 ngOnDestroy
ngOnDestroy Lifecycle Hooks This is called before the component is to be destroyed. So, this kind of hook is most useful for cleanup operations, like detaching event listeners or to unsubscribing from Observables.
Best Practices
- Always ngOnDestroy to close resources such as subscriptions otherwise create memory leaks.
- Perform here other cleanup tasks, such as removing event listeners or closing WebSocket connections.
4. Advanced Usage of Angular Hooks
- 4.1 Custom Hooks
As if Angular hadn’t already provided enough hooks out of the box, one can always create custom hooks, writing a series of functions to perform specific operations at different points of the life cycle. This can be done to standardize repetitive operations across different components.
- 4.2 Performance Optimization
Performance optimization, therefore comes in where you make sure that hooks like ngOnChanges, ngAfterViewChecked and ngDoCheck don’t get triggered unnecessarily. The developer can:
- Use memoization to prevent repeated work.
- Avoid putting complex logic on frequently called hooks to avoid performance degradation.
- 4.3 Error Handling
Angular Hooks also offer an opportunity to handle errors elegantly. For instance, you might use ngOnDestroy to clean up the resources nicely and, so, prevent potential crashes due to resource leaks.
5. Real-World Examples and Use Cases
- 5.1 Example 1: Managing Component State
In this example, we use ngOnInit to initialize a component’s state.
- The ngOnInit lifecycle hook is called only after the constructor of a component has been executed, and the component is fully initialized.
- In this example, an object with the title property is initialized to the data property and it’s created inside the ngInit method.
- This is a very basic example of providing a default state when a component is created. ngOnInit is a good place to put such initialization, because by this point the component is fully set up.
- 5.2 Example 2: Fetching Data on Component Initialization
We can make use of ngOnInit to call an API which can fetch some data whenever a component is initialized.
- In this sample example, the ngOnInit method is used to call an API via dataService when the component is initialized.
- They inject the DataService through a constructor, which is one of the regular Angular practices for dependency injection.
- In ngOnInit, there will be a call to the getData() method of dataService. The method of dataService is called there, and this returns an Observable. In the component, it subscribes to this Observable for the response data received – this answer is assigned to the data property.
- This will also show you how to get and display data when a component is loading.
- 5.3 Example 3: Cleanup and Resource Management
Using ngOnDestroy for unsubscription from Observables to prevent memory leaks.
- Here, in the SubscriptionComponent, it creates a subscription on an Observable. The ngOnInit call subscribes and the ngOnDestroy call is made for cleanup.
- The subscription property holds a copy of the result received when subscribing to the dataService.getData() method.
- The other lifecycle hook of Angular is ngOnDestroy, which is invoked just before the component gets destroyed.
- To prevent memory leaks, the method unsubscribe() is called in ngOnDestroy, allowing proper cleaning up of the subscription once a component is no longer needed. If any subscription is not unsubscribed, it can cause memory leaks because the Observable runs in the background.
6. Best Practices for Using Angular Hooks
- 6.1 Code Organization
Seamlessly organize hook logic so that the structure of your code could remain lucid and scalable. Complex logic should be kept separate methods and invoked by them in hooks.
- 6.2 Testing and Debugging
The hooks may be tested with Angular’s testing utilities simulating the lifecycle stage. Jasmine and Karma tools allow to invoke and test various stages of the life cycle.
- 6.3 Avoiding Common Pitfalls
- Overuse of ngDoCheck: Avoid this hook unless absolutely required because it has implications on performance.
- Not unsubscribing in ngOnDestroy:Always clean up the resources so that memory leaks do not occur.
7. Comparison with Other Frameworks
- 7.1 Angular Hooks vs. React Hooks
The management of the component lifecycle by hooks is shared between Angular and React, but React uses such hooks for state and side effect handling like useState, useEffect, whereas in Angular, it is special focus on a specifically defined lifecycle events.
- 7.2 Angular Hooks vs. Vue.js Lifecycle Hooks
Vue.js contains its set of lifecycle hooks (mounted, updated, destroyed) that is kind of parallel to the concept of hooks available in Angular, but, Angular in its totality offers more granular control over the different phases of a component’s lifecycle.
8. Conclusion
- 8.1 Summary of Key Points
Angular Hooks is thus an important mechanism in controlling the lifecycle of Angular components. It aids in organizing, optimizing, and then maintaining code with efficiency into applications.
- 8.2 Future Trends and Developments
As Angular matures, we can expect hooks to be better optimized in their use. We’d also expect better performance optimizations and tighter integration with features such as Signal that are expected in the coming updates on reactive programming.
Are you intrigued by the possibilities of AI? Let’s chat! We’d love to answer your questions and show you how AI can transform your industry. Contact Us