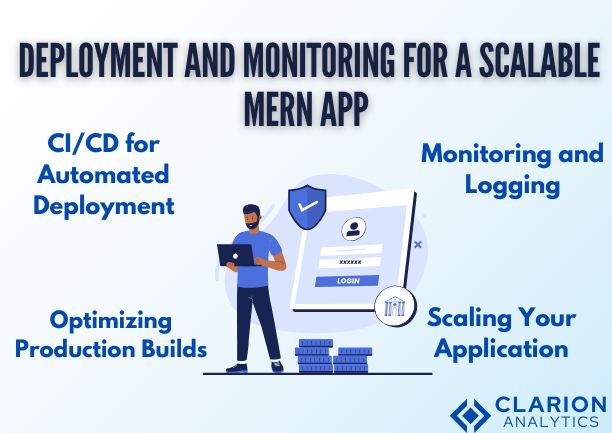
1. Structuring Your MERN Application for Maintainability
Project Structure Best Practices
A well-organized project structure enhances maintainability and scalability for MERN. Follow a modular approach, keeping separate folders for models, controllers, routes, and utilities in the backend. For the frontend, maintain components, hooks, and context in distinct directories.
Separation of Concerns
Ensure a clean separation between the frontend (React) and backend (Express/Node.js). Use a dedicated API layer for communication and avoid business logic in route handlers.
Using MVC Architecture
Implementing the Model-View-Controller (MVC) pattern keeps code manageable. Models handle data, controllers process logic, and views manage the UI. This ensures better scalability and ease of debugging.
Environment Variables Management
Sensitive data, such as API keys and database credentials, should be stored in .env
files and excluded from version control using .gitignore
. Use libraries like dotenv
to load environment variables securely.
2. Optimizing Backend Performance and Security
Efficient Database Queries
Optimize MongoDB queries by indexing frequently queried fields. Use aggregation pipelines and lean queries to fetch only necessary data.
Middleware Usage in Express
Utilize middleware for authentication, logging, and error handling. Tools like Morgan
help with request logging, while error-handling middleware ensures consistent API responses.
Rate Limiting and CORS Handling
Implement rate limiting using express-rate-limit
to prevent abuse. Configure CORS correctly to allow only necessary origins to access APIs.
JWT Authentication and Secure API Design
Use JWTs for authentication and refresh tokens for session management. Secure APIs with role-based access control and input validation using libraries like Joi
or Express Validator
.
3. Enhancing Frontend Performance in React
Component Reusability and Optimization
Utilize functional components and React hooks to write reusable code. Memoization with React.memo
and useCallback
prevents unnecessary re-renders.
State Management Best Practices
Choose the right state management solution. Context API is suitable for small apps, while Redux or Zustand is better for large-scale applications.
Lazy Loading and Code Splitting
Enhance page load speed by implementing dynamic imports using React’s React.lazy
and Suspense
. Code splitting helps reduce bundle size.
Optimized API Calls with React Query or SWR
Use libraries like React Query
or SWR
to manage API requests efficiently, reducing unnecessary re-fetching and improving caching mechanisms.
SEO and SSR in React Applications
Leverage Next.js for server-side rendering (SSR) to improve SEO and performance. Static site generation (SSG) can further optimize content delivery.
4. Deployment and Monitoring for a Scalable MERN
CI/CD for Automated Deployment
Implement continuous integration and deployment pipelines using GitHub Actions, Jenkins, or Docker to automate build and deployment processes for scalable mern
Optimizing Production Builds
Reduce bundle sizes with tree shaking and minification. Enable gzip compression to improve page load speed.
Monitoring and Logging
Use tools like Prometheus, Datadog, or LogRocket for real-time monitoring. Implement structured logging with Winston
or Morgan
.
Scaling Your Application
Scale your Node.js server using PM2 for process management. Implement horizontal scaling with load balancers and deploy on platforms like AWS, Vercel, or Heroku for better uptime in Mern
Are you intrigued by the possibilities of AI? Let’s chat! We’d love to answer your questions and show you how AI can transform your industry. Contact Us